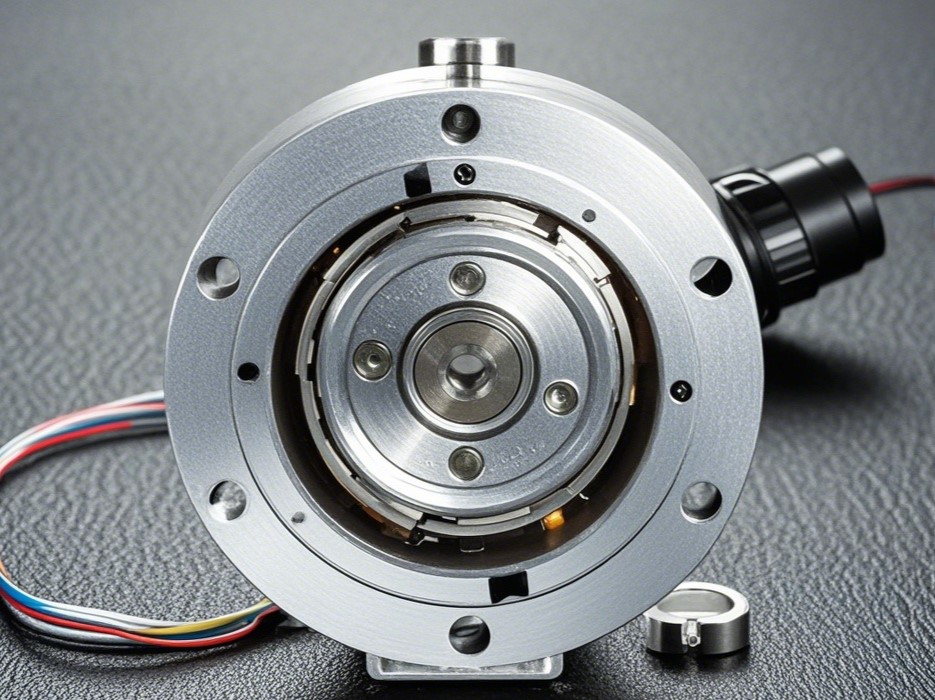
The ESP32Encoder library is a software library that enables efficient reading of rotation encoders by leveraging the pulse counter hardware peripheral of the ESP32. It offers numerous advantages such as high efficiency, low interrupt load, and multi-channel support, making it an ideal choice for handling rotation encoders in IoT and embedded projects.
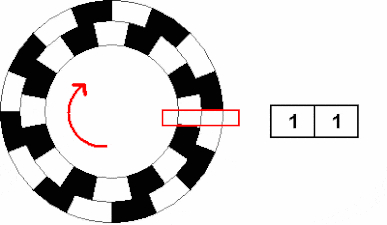
What is ESP32Encoder?
ESP32Encoder is an open-source library for the ESP32 microcontroller. It utilizes the built-in pulse counter (PCNT) hardware peripheral of the ESP32 to read the signals from rotation encoders. Compared to software polling methods, this library uses hardware interrupt handling, significantly reducing the CPU load and improving the reading accuracy and efficiency. It is especially suitable for applications that require real-time processing of rotation encoder data.
What can ESP32Encoder do?
ESP32Encoder is mainly used to read various types of rotation encoders, such as incremental encoders, and convert them into digital signals for subsequent processing by the microcontroller. This is crucial in many applications, such as:
-
Robot control: Precise control of the joint angles, speeds, and positions of robots.
-
Industrial automation: Monitoring and controlling the operating status of various mechanical equipment.
-
Instrumentation: Reading values on knobs or dials.
-
User interface: As a human-computer interaction method, such as volume adjustment, menu selection, etc.
The core functions and features of ESP32Encoder: -
Hardware acceleration: Utilizes the PCNT hardware peripheral of the ESP32 for counting, greatly reducing the software processing burden and improving efficiency and accuracy.
-
Low interrupt load: Only one interrupt is used to handle counter overflows, significantly reducing the CPU overhead of interrupt processing and ensuring stable system operation.
-
Multi-channel support: Supports up to 8 simultaneous encoders (for ESP32 and ESP32C2), and ESP32S3 supports 2.
-
Multiple counting modes: Supports three modes: full four-quadrant counting, half four-quadrant counting, and single-edge counting to adapt to different types of encoders and application requirements.
-
Internal weak pull-up/pull-down resistors: Configurable internal weak pull-up/pull-down resistors simplify the circuit design.
-
Configurable interrupt service CPU core: Allows users to specify the CPU core on which the interrupt service routine runs to avoid concurrency issues.
-
Hardware debouncing: Provides hardware debouncing functionality to effectively filter out jitter noise in the encoder signals. For encoders like KY-040 that are prone to jitter, external capacitors for debouncing and appropriate filtering parameters need to be set.
-
Easy to use: Offers a simple API interface for users to quickly integrate into their projects.
-
Good documentation: Provides detailed documentation generated by Doxygen for users to consult and learn.
Compatibility:
- Supports ESP32 and ESP32-C2 chips.
- ESP32-C3 is not supported as this chip does not have a pulse counter hardware.
- ESP32-S3 has only two PCNT modules, so only two hardware-accelerated encoders are supported.
Example
#include <ESP32Encoder.h>
ESP32Encoder encoder1(2, 4); // Connect the encoder using GPIO 2 and 4
void setup() {
Serial.begin(115200);
encoder1.setFilter(1023); // Set the maximum hardware debounce
encoder1.setCount(0); // Set the initial count to 0
}
void loop() {
long count = encoder1.getCount();
Serial.print("Encoder Count: ");
Serial.println(count);
delay(100);
}
Notes on KY-040 and similar encoders:
Switch-type encoders like KY-040 are prone to significant signal jitter and require external capacitors for debouncing (typically between 0.1uF and 2uF). Also, when using the ESP32Encoder library, setFilter(1023)
needs to be set to achieve the maximum hardware debouncing effect.
Conclusion:
The ESP32Encoder library is an efficient, easy-to-use, and powerful rotation encoder reading library. It fully utilizes the hardware resources of the ESP32, greatly simplifies the use of encoders, and improves system performance. Whether for simple applications or complex projects, ESP32Encoder can provide a reliable rotation encoder reading solution.
Project address: https://github.com/madhephaestus/ESP32Encoder